Reverbの自作2 all-pass filter
やや謎のall-pass filterにトライ。以前Phaserを作ったときにall-pass filterは作っているのだが、名前は同じでも構造も効果も違う。あまり名前にこだわらずにARIの解説の通りに作ることにする。前回のcomb filterと同じ遅延によるフィルターなのだが、all-pass filetrは周波数特性がフラットというのが特徴となっている。このフラットがどれぐらいを意味するのか不明だが、原理的には完全フラットということはあり得ないような気がする。まぁ作って判断するのが早い。
まずは、この通りプログラムをLADSPAで組んでみる。前回のcomb filterを修正するだけなので、数分で完成。むしろこのBlogを書いている時間が長い。
デルタ関数に適用すると、以下のようになった。1回目は反転して0.7がかけられている。2回目以降はプラスのままで、x1.0,x0.7,0.7 ・・・という具合になっている。
ホワイトノイズに適用してみた。確かにcombに比べれば平坦でフラットに近いかもしれないが、遅延時間を短くするとやはりくし形に近くなってくる。多少はマシという程度で考えるといいかもしれない。
これで下準備はできたので、次回から、これらを組合わせてリバーブとして機能させていく。
ソースは以下の通り。
sound programming 目次
all-pass filter ブロック図
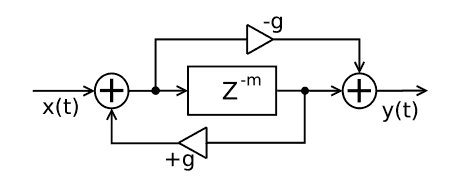
まずは、この通りプログラムをLADSPAで組んでみる。前回のcomb filterを修正するだけなので、数分で完成。むしろこのBlogを書いている時間が長い。

デルタ関数に適用すると、以下のようになった。1回目は反転して0.7がかけられている。2回目以降はプラスのままで、x1.0,x0.7,0.7 ・・・という具合になっている。

ホワイトノイズに適用してみた。確かにcombに比べれば平坦でフラットに近いかもしれないが、遅延時間を短くするとやはりくし形に近くなってくる。多少はマシという程度で考えるといいかもしれない。

これで下準備はできたので、次回から、これらを組合わせてリバーブとして機能させていく。
ソースは以下の通り。
LADSPA all-pass filter
|
sound programming 目次